Component technologies: The Innovative Components Powering
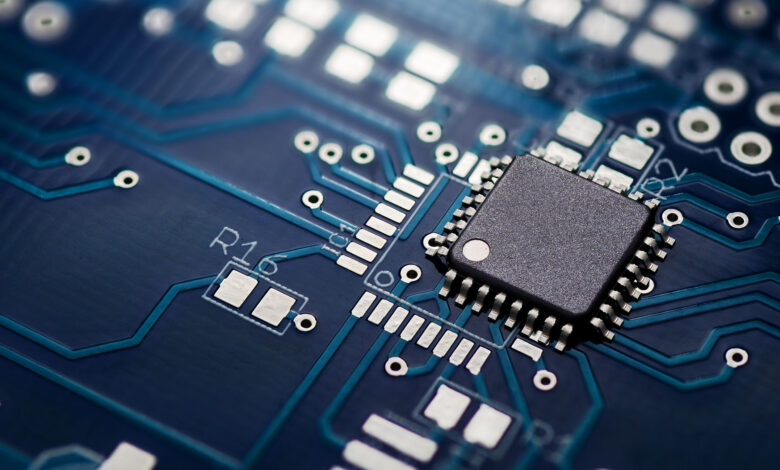
Component technologies are software architectures that structure applications as modular and reusable parts called components. These components work in isolation and can be independently deployed and managed. Component technologies have emerged as an efficient and flexible way to build complex applications by decomposing them into smaller interconnected parts.
This modularity enables greater flexibility, easier maintenance, and faster iteration as developers can focus on improving discrete parts of the application.
Component-based architectures have gained immense popularity over the past decade as web applications have grown increasingly complex. The ability to build software from pre-existing and pre-tested components boosts productivity, enhances quality, and enables greater consistency across applications. Component technologies like React, Angular, and Vue have transformed how modern front-ends are built. On the back end, component technologies like Spring and .NET Core aid in delivering scalable and robust systems. Overall, component technologies provide a powerful way to manage complexity and accelerate development.
History
The origins of component technologies can be traced back to the 1960s when modular programming techniques were first explored as a way to manage software complexity. The idea was to break down complex programs into smaller, reusable modules or components that could be independently developed, maintained, and reused across multiple projects.
In the 1990s, component-based software engineering gained popularity with the rise of object-oriented programming languages like C++ and Java. These languages made it easier to develop reusable software components through features like classes, interfaces, and inheritance. The middleware technologies CORBA and COM enabled components developed in different languages to communicate and integrate with each other.
The web also drove the evolution of component technologies in the 1990s, with the development of technologies like ActiveX, JavaBeans, and JavaScript libraries to build interactive web applications. Reusable UI components like buttons, menus, and widgets boosted developer productivity. The 2000s saw the rise of open-source JavaScript frameworks like React, Angular, and Vue that implemented a component-based architecture for structuring web applications.
Today, component technologies are pervasive across application development. Modern frameworks provide extensive libraries of reusable UI components and tools to develop custom components. Component-based development and distributed component architectures have become the state-of-the-art in building modular, scalable applications.
Benefits
Component-based development offers some key advantages compared to traditional monolithic application development:
Improved reusability – Components are designed to be reusable across multiple applications. Once a component is created, it can be reused repeatedly without needing to be rewritten. This saves significant development time and cost.
Improved maintainability – Because components are decoupled from each other, they can be maintained in isolation without affecting the rest of the system. This makes locating and fixing bugs much easier. Additionally, updating a component in one place improves it across all applications using that component.
Improved flexibility – Components can be more easily customized, configured, and extended to meet changing requirements. New capabilities can be added by creating new components or swapping existing ones. The modular architecture makes applications more adaptable.
By leveraging these advantages, component-based development enables faster, lower-cost development and easier maintenance of complex applications. Modularity and encapsulation promote good software engineering principles. Components can also enable the assembly of applications from pre-built parts to accelerate delivery.
Types of Component Technologies
Client-side Components
Client-side components are pieces of code that run on the user’s web browser, handling the presentation layer and front-end logic of web applications. Examples include JavaScript frameworks like React, Angular and Vue which break down complex UIs into modular, reusable components. These provide benefits like improved code organization, separation of concerns, and faster rendering through virtual DOM diffing. Client-side components allow you to divide UI code into self-contained units that are easier to build, update, and maintain. They also enable smoother UI interactions without full-page reloads.
Server-side Components
Server-side components are pieces of code that run on the web server, generating the HTML views sent to the client. They handle backend application logic like data access and processing. Examples are view components in frameworks like ASP.NET, JSF, and Spring MVC. These encapsulate code to render UI sections server-side before sending the final HTML to the browser. Benefits include reduced client-side code, the ability to leverage server capabilities, and SEO-friendliness. However, they can result in slower page loads due to full page refreshes.
Database Components
Database components refer to reusable modules that encapsulate database access logic. Examples are ORM frameworks like Hibernate and entity frameworks. These provide out-of-the-box capabilities for mapping object-oriented domain models to database tables. They handle querying, CRUD operations, schema generation and other data persistence concerns. Benefits include increased productivity and maintainability by abstracting away SQL and database access code. Database components promote code reuse and allow switching between different database systems with lower effort.
Frameworks
Component frameworks provide structure and organization for building component-based user interfaces. They abstract away much of the complexity in handling component communication, state management, rendering, and more. Some of the most popular JavaScript frameworks for building component-based web applications include:
- React – Created by Facebook and one of the most widely used front-end libraries today. React uses a declarative, component-focused approach to build fast and dynamic web apps. Key features include its use of virtual DOM, one-way data flow, and JSX syntax. React components are reusable, composable building blocks that manage their own state.
- Angular – Developed by Google, Angular is a TypeScript-based framework using an MVC architecture. It provides many out-of-the-box features like data binding, dependency injection, and routing.
- Vue – An increasingly popular progressive framework created by Evan You. Vue combines the best of Angular and React with an incremental adoption approach. It uses a template syntax similar to Angular and reactive components like React. Vue is flexible and lightweight yet features rich composability and tooling.
- NET Core – For building component-based web apps using C#, .NET Core provides Blazor. Blazor lets you build UI components using C# instead of JavaScript. It features server-side and client-side rendering options and integrates seamlessly with .NET Core APIs.
These frameworks maximize productivity and maintainability by encouraging modular component architecture. They provide the essential tools for building complex component-based UIs at scale while handling much of the complexity behind the scenes.
Component Design
Designing effective components requires focusing on key principles like encapsulation, clear interfaces, and composability.
Encapsulation is crucial for components. Each component should encapsulate its data and behavior so it can operate independently. Components should expose a minimal API surface, only revealing what other components need to interact with it. This allows changing component internals without affecting the larger system.
Well-defined interfaces enable components to cleanly interact. Interfaces describe what data enters and exits a component. Standard interfaces like props and events in React encourage consistency. Components with clear interfaces are more reusable, portable, and adaptable.
Composability means components work synergistically. This allows flexible UI construction.
Following these principles results in robust, modular components. They can be reused across projects, accelerating development. Loose coupling between components also allows easier testing, maintenance and scaling of codebases. Investing in thoughtful component design upfront pays dividends through increased productivity down the line.
Development
Developing reusable components requires more deliberate planning and effort up front, but saves time in the long run. Here are some best practices to follow when building components:
Building Components
- Components should be built with reusability and flexibility in mind from the start. They need to work in different contexts and scenarios.
- Strive for loose coupling between components so they can be used independently. Avoid overly tight dependencies between components.
- Build components in a modular fashion, breaking large components into smaller sub-components. This increases composability.
- Component logic should be separate from the visual presentation. Use CSS and HTML for styling.
- Follow a consistent structure and API design across components. This makes them easier to understand and implement.
Testing Components
- Thoroughly unit test components to ensure they function as intended. Refactor code that is difficult to test.
- Perform integration testing with other components to find issues early. Verify components work together properly.
- Do cross-browser and device testing to check responsiveness and compatibility. Components should work across environments.
Documenting Components
Components should have clear documentation on usage, input and output, dependencies, examples, and more. This helps other developers use them properly.
- Use examples and demo apps to show components in action. Make documentation easy to follow.
- Document how components should be extended or customized by other developers. Explain any limitations.
Versioning Components
- Use semantic versioning to manage releases of component libraries and APIs.
- Maintain clear CHANGELOGs explaining changes, additions, and deprecations between versions.
- Avoid breaking changes in minor/patch releases. Reserve for major versions.
- Ensure proper use of versioning for dependencies between components and integrations.
Following these practices during development produces high-quality reusable components that save significant time over the long term. Investing in robust components pays dividends across projects and products.
Implementation
Implementing component-based architectures can be challenging due to the increased complexity of breaking down an application into separate components. Here are some key considerations for implementation:
- Integration– Component granularity and clear interfaces are crucial for smooth integration between components. Avoid tight coupling between components. Services and messaging help connect components that don’t reference each other directly.
- Dependency Management – Track all dependencies between components to avoid conflicts. Manage dependencies carefully through an ecosystem like npm. Watch out for dependency hell resulting from conflicting versions.
- Updating – Have a strategy for keeping components up-to-date, like semantic versioning. Make components backward compatible when possible. Test and validate components thoroughly when updating.
- Deployment – Components may be deployed independently. Plan how to deploy updates without downtime. Use techniques like blue-green deployment, feature toggles, and canary releases.
- Monitoring – Monitor components in production for performance, errors, and dependencies. Quickly detect issues with tracing, logging, and analytics. Have visibility across all components.
- Organization – Structure teams, code, and architecture around components. Enforce clear ownership and responsibilities for each component. Align team workflows with component boundaries.
- Scalability – Design stateless components that can scale horizontally. Distribute load across component instances. Watch for shared components that may bottleneck at scale.
- Security – Limit access between components based on least privilege. Authenticate and authorize at the component level. Validate inputs and handle errors gracefully within components.
With careful planning and execution, component-based architectures can greatly improve development velocity and application quality. But the increased complexity requires upfront investment to avoid pitfalls.
Trends
The use of component technologies continues to grow rapidly as developers recognize the benefits of modular code and reusable UI elements. Some key trends include:
Increasing Adoption
- Component architectures are being adopted more widely across organizations and industries. Many major tech companies now rely extensively on component libraries and frameworks.
- The popularity of JavaScript frameworks like React, Vue, and Angular that utilize components has driven increased component adoption. These frameworks make it easier to build UIs from reusable components.
- Open-source component libraries allow developers to easily share and consume components. This facilitates component uptake by removing the need to build common components from scratch.
New Frameworks and Tools
- New frameworks and component libraries continue to emerge, providing developers more choice. Examples include Svelte, Lit, and Mantine.
- Tools are making it easier to develop, document, test, and share component libraries internally and externally. Storybook and Bit are two popular component dev tools.
Cross-Platform Components
- Components originally designed for the web are now being adapted for use in cross-platform apps. React Native enables using React components in mobile apps.
- Flutter and other cross-platform frameworks rely on reusable components that can render across web, mobile, and desktop.
- The ability to reuse UI components across platforms makes cross-platform development more efficient.
Conclusion
Summary
Component technologies have made enormous strides in recent years, transforming the way software is designed and built. By breaking complex applications into reusable components, developers gain greater flexibility, efficiency, and maintainability. Component architectures such as microservices promote loose coupling and modularity, enabling more scalable and adaptable systems.
Popular frameworks like React, Vue, and Angular provide robust component models for building interactive user interfaces. On the backend, component technologies like Spring and .NET Core foster component-based development. Containerization platforms like Docker have also been instrumental in componentizing infrastructure and deployments.
The core concepts behind components – encapsulation, abstraction, and separation of concerns – have proven tremendously beneficial across the software landscape. Component-based and service-oriented architectures will only grow in popularity as we build more complex cloud-native applications.
Future Outlook
Looking ahead, we will likely see component technologies continue to evolve and expand. Trends like Web Components will push reusable components into the browser, while serverless computing could revolutionize backend components.
As applications rely more on AI/ML capabilities, components embedded with intelligent services will emerge. There may also be greater integration of UI components with backend business logic and data through full-stack frameworks.
To realize componentization’s full potential, organizations will need to invest in proper governance, testing, documentation, and DevOps tooling. With the right foundations in place, component technologies promise to enable developers to build, connect, and maintain complex systems more efficiently than ever before. The future is bright for the world of interconnected componentized architectures.